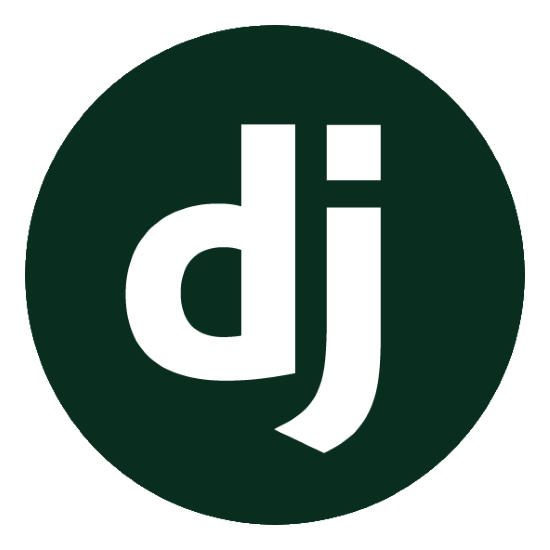
I don't understand the purpose of this library. The article makes it sound like the problem is circular dependencies, which is not something that is addressed by the library in any way. Deleting and regenerating all the migrations instead of squashing is something I’ve done before (it often generates a much simpler squashed migration than squashmigrations does), but it is very much a manual process, for example you must review the generated migration and bring in any runpython/runsql operations, because makemigrations won’t do that. And the replaces
directive built in to Django handles fixing the history across environments already.
Some more examples of why piracy is a service problem