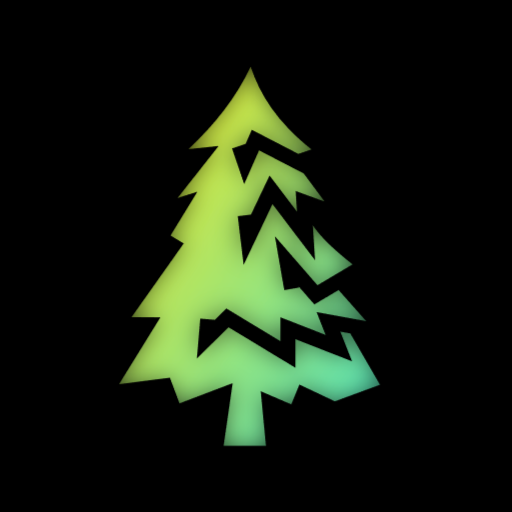
When you substitute the first two question marks with ##, the answer already doesn’t match the input string, so you can throw away 1M of the combinations that don’t fit.
You know I figured I was already doing that, but printing your example shows I was not. I also added some logic to the other end since 1,2,2 needs a space of 7 and if the check is all dots and I only have 6 chars left, I know it can't fit.
still taking a long time for the real data so it must be something inefficient in my code then, rather than the method.
Also, while you’re at it, avoid generic type annotations
Good point. Recently figured that one out, still not automatic as you can see.
Thanks for the pointers.
This felt ... too simple. I think the hardest part of part two for me was reading comprehension. My errors were typically me not reading exactly was there.
Python